GDEXTENSION 公式サンプルの Windows 及び WEB(HTML5)向けにビルド
本稿は GDEXTENSION の WEB エクスポートを試した際のメモの共有を意図した、一連の記事の3番目。1番目から順に読まれていくことを想定している。
対象としている Godot エンジンのバージョンは 4.2.1。 emscripten のバージョンは 3.1.39。
前稿では、emscripten をインストールし WEB(HTML5)用ビルド環境を構築した。また、Godot エンジンの WEB(HTML5)向けエクスポートテンプレートのビルドを試した。
本稿では公式にある GDEXTENSION サンプルを、Windows 向け及び WEB(HTML5)向けにビルドを行う。
GDEXTENSION サンプルのビルド
ソースコード
今回試す GDEXTENSION サンプルは Godot エンジンの公式のチュートリアルのもので、Godot GDEXTENSION build 環境構築のまとめ(その3) で試したものと同じ。
ソースコードは以下の4つ。
- gdexample.cpp
- gdexample.h
- register_types.cpp
- register_types.h
gdexample.h
#ifndef GDEXAMPLE_H
#define GDEXAMPLE_H
#include <godot_cpp/classes/sprite2d.hpp>
namespace godot {
class GDExample : public Sprite2D {
GDCLASS(GDExample, Sprite2D)
private:
double time_passed;
protected:
static void _bind_methods();
public:
GDExample();
~GDExample();
void _process(double delta);
};
}
#endif
gdexample.cpp
#include "gdexample.h"
#include <godot_cpp/core/class_db.hpp>
using namespace godot;
void GDExample::_bind_methods() {
}
GDExample::GDExample() {
// Initialize any variables here.
time_passed = 0.0;
}
GDExample::~GDExample() {
// Add your cleanup here.
}
void GDExample::_process(double delta) {
time_passed += delta;
Vector2 new_position = Vector2(10.0 + (10.0 * sin(time_passed * 2.0)), 10.0 + (10.0 * cos(time_passed * 1.5)));
set_position(new_position);
}
register_types.h
#ifndef GDEXAMPLE_REGISTER_TYPES_H
#define GDEXAMPLE_REGISTER_TYPES_H
void initialize_example_module();
void uninitialize_example_module();
#endif // GDEXAMPLE_REGISTER_TYPES_H
register_types.cpp
#include "register_types.h"
#include "gdexample.h"
#include <gdextension_interface.h>
#include <godot_cpp/core/defs.hpp>
#include <godot_cpp/core/class_db.hpp>
#include <godot_cpp/godot.hpp>
using namespace godot;
void initialize_example_module(ModuleInitializationLevel p_level) {
if (p_level != MODULE_INITIALIZATION_LEVEL_SCENE) {
return;
}
ClassDB::register_class<GDExample>();
}
void uninitialize_example_module(ModuleInitializationLevel p_level) {
if (p_level != MODULE_INITIALIZATION_LEVEL_SCENE) {
return;
}
}
extern "C" {
// Initialization.
GDExtensionBool GDE_EXPORT example_library_init(GDExtensionInterfaceGetProcAddress p_get_proc_address, const GDExtensionClassLibraryPtr p_library, GDExtensionInitialization *r_initialization) {
godot::GDExtensionBinding::InitObject init_obj(p_get_proc_address, p_library, r_initialization);
init_obj.register_initializer(initialize_example_module);
init_obj.register_terminator(uninitialize_example_module);
init_obj.set_minimum_library_initialization_level(MODULE_INITIALIZATION_LEVEL_SCENE);
return init_obj.init();
}
}
Sconstruct
SConstruct ファイルは以下のものを使う。
C:\dev\gdextension_sample\sample_01\SConstruct
#!/usr/bin/env python
import os
import sys
import SCons.Util
project_name = 'libgdexample'
env = SConscript('./godot-cpp-4.2.1-stable/SConstruct')
print('build target:', type(env['target']), env['target'])
print("env['CCFLAGS']:", type(env['CCFLAGS']), env['CCFLAGS'])
print("env['CFLAGS']:", type(env['CFLAGS']), env['CFLAGS'])
print("env['CXXFLAGS']:", type(env['CXXFLAGS']), env['CXXFLAGS'])
print("env['CPPDEFINES']:", type(env['CPPDEFINES']), env['CPPDEFINES'])
print("env['LINKFLAGS']:", type(env['LINKFLAGS']), env['LINKFLAGS'])
print("env['CPPPATH']:")
for i in env['CPPPATH']:
print(" ",type(i), i)
print("env['LIBS']:")
for i in env['LIBS']:
print(" ",type(i), i)
if env['platform'] == "windows" and env['use_mingw'] == True and env['arch'] == "x86_64":
# strip -fno-exceptions from $CXXFLAGS
env['CXXFLAGS'] = SCons.Util.CLVar(str(env['CXXFLAGS']).replace("-fno-exceptions", ""))
print("env['CXXFLAGS']", env['CXXFLAGS'])
env.Append(LINKFLAGS = ['-static-libgcc', '-static-libstdc++', '-static', '-pthread'])
elif env['platform'] == "web" and env['arch'] == "wasm32":
# strip -fno-exceptions from $CXXFLAGS
env['CXXFLAGS'] = SCons.Util.CLVar(str(env['CXXFLAGS']).replace("-fno-exceptions", ""))
print("env['CXXFLAGS']", env['CXXFLAGS'])
else:
print("not suppoted conbination!")
print("platform",env["platform"])
print("use_mingw",env["use_mingw"])
print("use_clang_cl",env["use_clang_cl"])
print("use_static_cpp",env["use_static_cpp"])
print("android_api_level",env["android_api_level"])
print("ios_simulator",env["ios_simulator"])
print("arch",env["arch"])
exit()
env.Append(CPPPATH=["src/"])
print("env['CPPPATH']", env['CPPPATH'])
for i in env['CPPPATH']:
print(" ",type(i), i)
sources = Glob("src/*.cpp")
print("CPPDEFINES",env['CPPDEFINES'])
library = env.SharedLibrary(
"project/bin/{}{}{}".format(project_name,env["suffix"], env["SHLIBSUFFIX"]),
source=sources,
)
Default(library)
この SConstruct ファイルは以前 MinGW-w64 用に作成したものに対し、以下の部分を付け加えている。
elif env['platform'] == "web" and env['arch'] == "wasm32":
# strip -fno-exceptions from $CXXFLAGS
env['CXXFLAGS'] = SCons.Util.CLVar(str(env['CXXFLAGS']).replace("-fno-exceptions", ""))
print("env['CXXFLAGS']", env['CXXFLAGS'])
これは、WEB(HTML5)ターゲットビルド用の差分であり、platform=web、arch=wasm32 の組み合わせ時にビルドを許可しするとともに、コンパイルオプションの -fno-exceptions を剥ぐ。
この SConstruct を用いることで、MinGW-w64(Windows ターゲット) および emscripten(WEB ターゲット)の両方に対応する。
ディレクトリ配置
“C:\dev\gdextension_sample\sample_01” というディレクトリに gdextension のサンプルを作成することとする。ソースコードファイルと SConstruct ファイルを以下のように設置する。
C:\dev\gdextension_sample\sample_01 │ SConstruct │ └─src gdexample.cpp gdexample.h register_types.cpp register_types.h
godot-cpp をプロジェクトのサブモジュールとすべく、取得する。
Microsoft Windows [Version 10.0.22631.2861] (c) Microsoft Corporation. All rights reserved. c:\Users\user>mingw64.bat c:\dev>cd gdextension_sample\sample_01 c:\dev\gdextension_sample\sample_01>git clone --depth 1 https://github.com/godotengine/godot-cpp.git -b godot-4.2.1-stable godot-cpp-4.2.1-stable
この結果ディレクトリ構造は以下のようになる。
C:\dev\gdextension_sample\sample_01 │ │ SConstruct │ ├─godot-cpp-4.2.1-stable │ ※多数のファイル │ └─src gdexample.cpp gdexample.h register_types.cpp register_types.h
以上でビルドの準備は整った。これを Windows ターゲット/WEB(HTML5)ターゲット、デバッグビルド/リリースビルドの組み合わせで合計4回ビルドを行う。
Windows ターゲット、WEB(HTML5)ターゲットではコマンドプロンプトを別に開き、それぞれの環境設定用バッチファイルを実行する必要がある。
Windows プラットフォーム向けビルド
環境設定
新しいコマンドプロンプトを開き以下を実行する。
Microsoft Windows [Version 10.0.22631.3007] (c) Microsoft Corporation. All rights reserved. C:\Users\user>.\mingw64.bat c:\dev>cd gdextension_sample\sample_01
リリースビルド
Windows ターゲットのリリースビルドは以下を実行する。
c:\dev\gdextension_sample\sample_01>scons platform=windows use_mingw=yes target=template_release
SConstruct に環境変数を吐き出す print 分を仕込んである。Windows ターゲットのリリースビルドの場合は以下のような出力になる。
scons: Reading SConscript files ... Auto-detected 12 CPU cores available for build parallelism. Using 11 cores by default. You can override it with the -j argument. Building for architecture x86_64 on platform windows build target: <class 'str'> template_release env['CCFLAGS']: <class 'SCons.Util.CLVar'> -Wwrite-strings -O3 -fvisibility=hidden env['CFLAGS']: <class 'SCons.Util.CLVar'> env['CXXFLAGS']: <class 'SCons.Util.CLVar'> -fno-exceptions -std=c++17 env['CPPDEFINES']: <class 'collections.deque'> deque(['WINDOWS_ENABLED', 'NDEBUG']) env['LINKFLAGS']: <class 'SCons.Util.CLVar'> -Wl,--no-undefined -static -static-libgcc -static-libstdc++ -s -fvisibility=hidden env['CPPPATH']: <class 'SCons.Node.FS.Dir'> godot-cpp-4.2.1-stable\gdextension <class 'SCons.Node.FS.Dir'> godot-cpp-4.2.1-stable\include <class 'SCons.Node.FS.Dir'> godot-cpp-4.2.1-stable\gen\include env['LIBS']: <class 'SCons.Node.FS.File'> godot-cpp-4.2.1-stable\bin\libgodot-cpp.windows.template_release.x86_64.a env['CXXFLAGS'] -std=c++17 env['CPPPATH'] [<SCons.Node.FS.Dir object at 0x000001BC1E3309D0>, <SCons.Node.FS.Dir object at 0x000001BC1F545440>, <SCons.Node.FS.Dir object at 0x000001BC1E32E890>, 'src/'] <class 'SCons.Node.FS.Dir'> godot-cpp-4.2.1-stable\gdextension <class 'SCons.Node.FS.Dir'> godot-cpp-4.2.1-stable\include <class 'SCons.Node.FS.Dir'> godot-cpp-4.2.1-stable\gen\include <class 'str'> src/ CPPDEFINES deque(['WINDOWS_ENABLED', 'NDEBUG']) scons: done reading SConscript files. scons: Building targets ...
ビルドが成功すると以下の生成物を得る。
C:\dev\gdextension_sample\sample_01\project\bin\libgdexample.windows.template_release.x86_64.dll
デバッグビルド
Windows ターゲットのデバッグビルドは以下を実行する。
c:\dev\gdextension_sample\sample_01>scons platform=windows use_mingw=yes target=template_debug
SConstruct に仕込んだ環境変数 printの、Windows ターゲットデバッグビルドの出力は以下のようになる。
scons: Reading SConscript files ... Auto-detected 12 CPU cores available for build parallelism. Using 11 cores by default. You can override it with the -j argument. Building for architecture x86_64 on platform windows build target: <class 'str'> template_debug env['CCFLAGS']: <class 'SCons.Util.CLVar'> -Wwrite-strings -O2 -fvisibility=hidden env['CFLAGS']: <class 'SCons.Util.CLVar'> env['CXXFLAGS']: <class 'SCons.Util.CLVar'> -fno-exceptions -std=c++17 env['CPPDEFINES']: <class 'collections.deque'> deque(['HOT_RELOAD_ENABLED', 'WINDOWS_ENABLED', 'DEBUG_ENABLED', 'DEBUG_METHODS_ENABLED', 'NDEBUG']) env['LINKFLAGS']: <class 'SCons.Util.CLVar'> -Wl,--no-undefined -static -static-libgcc -static-libstdc++ -s -fvisibility=hidden env['CPPPATH']: <class 'SCons.Node.FS.Dir'> godot-cpp-4.2.1-stable\gdextension <class 'SCons.Node.FS.Dir'> godot-cpp-4.2.1-stable\include <class 'SCons.Node.FS.Dir'> godot-cpp-4.2.1-stable\gen\include env['LIBS']: <class 'SCons.Node.FS.File'> godot-cpp-4.2.1-stable\bin\libgodot-cpp.windows.template_debug.x86_64.a env['CXXFLAGS'] -std=c++17 env['CPPPATH'] [<SCons.Node.FS.Dir object at 0x000001B8CAFD42B0>, <SCons.Node.FS.Dir object at 0x000001B8CC1C5440>, <SCons.Node.FS.Dir object at 0x000001B8CAFD5350>, 'src/'] <class 'SCons.Node.FS.Dir'> godot-cpp-4.2.1-stable\gdextension <class 'SCons.Node.FS.Dir'> godot-cpp-4.2.1-stable\include <class 'SCons.Node.FS.Dir'> godot-cpp-4.2.1-stable\gen\include <class 'str'> src/ CPPDEFINES deque(['HOT_RELOAD_ENABLED', 'WINDOWS_ENABLED', 'DEBUG_ENABLED', 'DEBUG_METHODS_ENABLED', 'NDEBUG']) scons: done reading SConscript files. scons: Building targets ...
ビルドが成功すると以下の生成物を得る。
C:\dev\gdextension_sample\sample_01\project\bin\libgdexample.windows.template_debug.x86_64.dll
WEB(HTML5)向けビルド
環境設定
Microsoft Windows [Version 10.0.22631.3007] (c) Microsoft Corporation. All rights reserved. C:\Users\kano>.\emsdk c:\dev>cd gdextension_sample\sample_01
リリースビルド
WEB(HTML5)ターゲットのリリースビルドは以下を実行する。
c:\dev\gdextension_sample\sample_01>scons platform=web target=template_release
SConstruct に仕込んだ環境変数 printの、WEB(HTML5)ターゲットリリースビルドの出力は以下のようになる。
scons: Reading SConscript files ... Auto-detected 12 CPU cores available for build parallelism. Using 11 cores by default. You can override it with the -j argument. Building for architecture wasm32 on platform web build target: <class 'str'> template_release env['CCFLAGS']: <class 'SCons.Util.CLVar'> -s USE_PTHREADS=1 -O3 -fvisibility=hidden env['CFLAGS']: <class 'SCons.Util.CLVar'> env['CXXFLAGS']: <class 'SCons.Util.CLVar'> -fno-exceptions -std=c++17 env['CPPDEFINES']: <class 'collections.deque'> deque(['WEB_ENABLED', 'UNIX_ENABLED', 'NDEBUG']) env['LINKFLAGS']: <class 'SCons.Util.CLVar'> -s USE_PTHREADS=1 -s SIDE_MODULE=1 -s -fvisibility=hidden env['CPPPATH']: <class 'SCons.Node.FS.Dir'> godot-cpp-4.2.1-stable\gdextension <class 'SCons.Node.FS.Dir'> godot-cpp-4.2.1-stable\include <class 'SCons.Node.FS.Dir'> godot-cpp-4.2.1-stable\gen\include env['LIBS']: <class 'SCons.Node.FS.File'> godot-cpp-4.2.1-stable\bin\libgodot-cpp.web.template_release.wasm32.a env['CXXFLAGS'] -std=c++17 env['CPPPATH'] [<SCons.Node.FS.Dir object at 0x000001ECCC5B7E00>, <SCons.Node.FS.Dir object at 0x000001ECCF6AEDB0>, <SCons.Node.FS.Dir object at 0x000001ECCC5B9360>, 'src/'] <class 'SCons.Node.FS.Dir'> godot-cpp-4.2.1-stable\gdextension <class 'SCons.Node.FS.Dir'> godot-cpp-4.2.1-stable\include <class 'SCons.Node.FS.Dir'> godot-cpp-4.2.1-stable\gen\include <class 'str'> src/ CPPDEFINES deque(['WEB_ENABLED', 'UNIX_ENABLED', 'NDEBUG']) scons: done reading SConscript files. scons: Building targets ...
ビルドが成功すると以下の生成物を得る。
C:\dev\gdextension_sample\sample_01\project\bin\libgdexample.web.template_release.wasm32.wasm
デバッグビルド
WEB(HTML5)ターゲットのデバッグビルドは以下を実行する。
c:\dev\gdextension_sample\sample_01>scons platform=web target=template_debug
SConstruct に仕込んだ環境変数 printの、WEB(HTML5)ターゲットデバッグビルドの出力は以下のようになる。
scons: Reading SConscript files ... Auto-detected 12 CPU cores available for build parallelism. Using 11 cores by default. You can override it with the -j argument. Building for architecture wasm32 on platform web build target: <class 'str'> template_debug env['CCFLAGS']: <class 'SCons.Util.CLVar'> -s USE_PTHREADS=1 -O2 -fvisibility=hidden env['CFLAGS']: <class 'SCons.Util.CLVar'> env['CXXFLAGS']: <class 'SCons.Util.CLVar'> -fno-exceptions -std=c++17 env['CPPDEFINES']: <class 'collections.deque'> deque(['HOT_RELOAD_ENABLED', 'WEB_ENABLED', 'UNIX_ENABLED', 'DEBUG_ENABLED', 'DEBUG_METHODS_ENABLED', 'NDEBUG']) env['LINKFLAGS']: <class 'SCons.Util.CLVar'> -s USE_PTHREADS=1 -s SIDE_MODULE=1 -s -fvisibility=hidden env['CPPPATH']: <class 'SCons.Node.FS.Dir'> godot-cpp-4.2.1-stable\gdextension <class 'SCons.Node.FS.Dir'> godot-cpp-4.2.1-stable\include <class 'SCons.Node.FS.Dir'> godot-cpp-4.2.1-stable\gen\include env['LIBS']: <class 'SCons.Node.FS.File'> godot-cpp-4.2.1-stable\bin\libgodot-cpp.web.template_debug.wasm32.a env['CXXFLAGS'] -std=c++17 env['CPPPATH'] [<SCons.Node.FS.Dir object at 0x00000167BC38DB70>, <SCons.Node.FS.Dir object at 0x00000167BD631960>, <SCons.Node.FS.Dir object at 0x00000167BC38D1F0>, 'src/'] <class 'SCons.Node.FS.Dir'> godot-cpp-4.2.1-stable\gdextension <class 'SCons.Node.FS.Dir'> godot-cpp-4.2.1-stable\include <class 'SCons.Node.FS.Dir'> godot-cpp-4.2.1-stable\gen\include <class 'str'> src/ CPPDEFINES deque(['HOT_RELOAD_ENABLED', 'WEB_ENABLED', 'UNIX_ENABLED', 'DEBUG_ENABLED', 'DEBUG_METHODS_ENABLED', 'NDEBUG']) scons: done reading SConscript files. scons: Building targets ...
ビルドが成功すると以下の生成物を得る。
C:\dev\gdextension_sample\sample_01\project\bin\libgdexample.web.template_debug.wasm32.wasm
Godot エンジンプロジェクトの作成
Godot エンジンプロジェクトの作成
先ず Godot エンジンプロジェクト用のディレクトリを作成する。
Microsoft Windows [Version 10.0.22631.3007] (c) Microsoft Corporation. All rights reserved. C:\Users\user>.\mingw64.bat c:\dev>cd demos c:\dev\demos>mkdir demo_05 c:\dev\demos>cd demo_05
空の Godot エンジンプロジェクトを作成。
c:\dev\demos\demo_05>copy nul .\project.godot
bin ディレクトリを作成し、GDEXTENSION のダイナミックリンクライブラリを設置する。
c:\dev\demos\demo_05>mkdir bin c:\dev\demos\demo_05>cd bin c:\dev\demos\demo_05\bin>copy C:\dev\gdextension_sample\sample_01\project\bin\*.dll . c:\dev\demos\demo_05\bin>copy C:\dev\gdextension_sample\sample_01\project\bin\*.wasm .
c:\dev\demos\demo_05\bin ディレクトリに以下のような gdextension ファイルを作成する。
c:\dev\demos\demo_05\bin\gdexample.gdextension
[configuration]
entry_symbol = "example_library_init"
compatibility_minimum = "4.1"
[libraries]
windows.debug.x86_64 = "res://bin/libgdexample.windows.template_debug.x86_64.dll"
windows.release.x86_64 = "res://bin/libgdexample.windows.template_release.x86_64.dll"
web.debug.wasm32 = "res://bin/libgdexample.web.template_debug.wasm32.wasm"
web.release.wasm32 = "res://bin/libgdexample.web.template_release.wasm32.wasm"
ここまでの手順で、ディレクトリ構成は以下のようになる。
C:\dev\demos\demo_05 │ project.godot │ └─bin\ gdexample.gdextension libgdexample.windows.template_debug.x86_64.dll" libgdexample.windows.template_release.x86_64.dll" libgdexample.web.template_debug.wasm32.wasm" libgdexample.web.template_release.wasm32.wasm"
Godot エンジンプロジェクトを起動する。
c:\dev\demos\demo_05>godot.windows.editor.x86_64.exe -e .
名無しのプロジェクトが起動する。
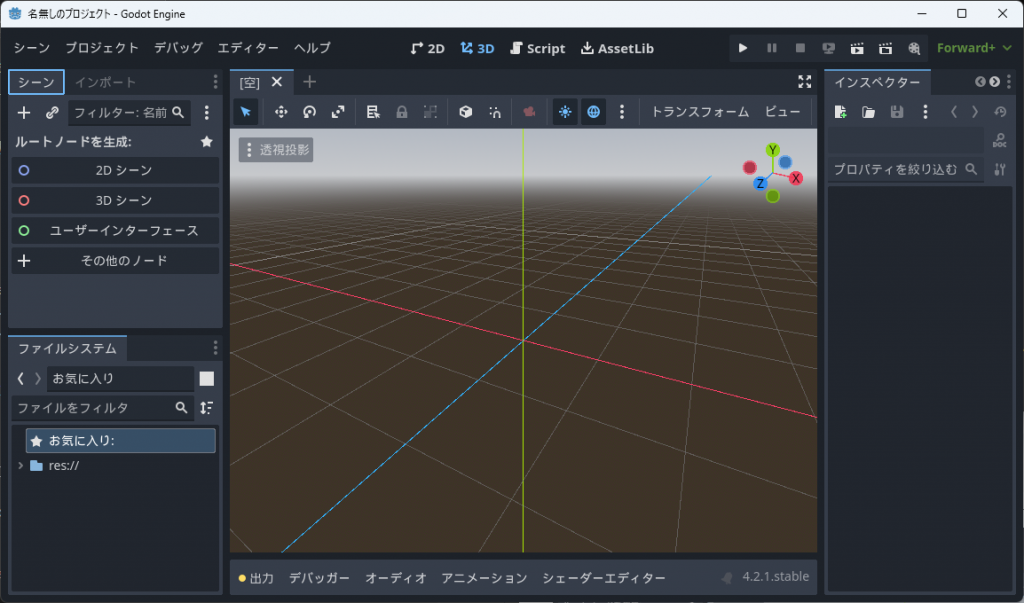
GDEXTENSION が読み込まれている場合は、左下のリソースツリーに gdexample.gdextension が存在する。
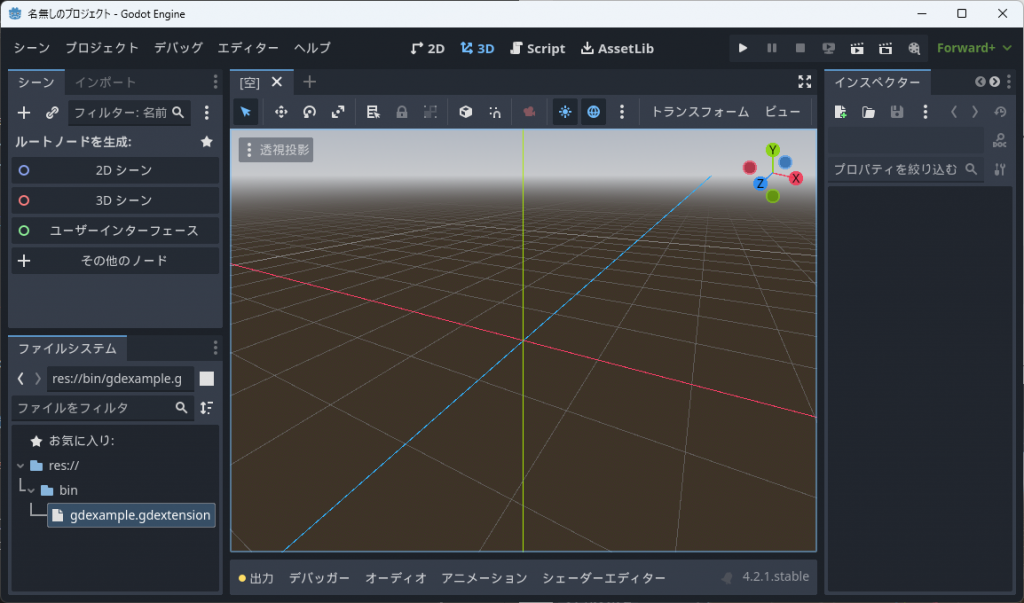
res:// └─bin/ gdexample.gdextension
先ずシーンを設定する。メニューの「シーン」を選ぶとメニューが現れるので「新規シーン」を選択する。
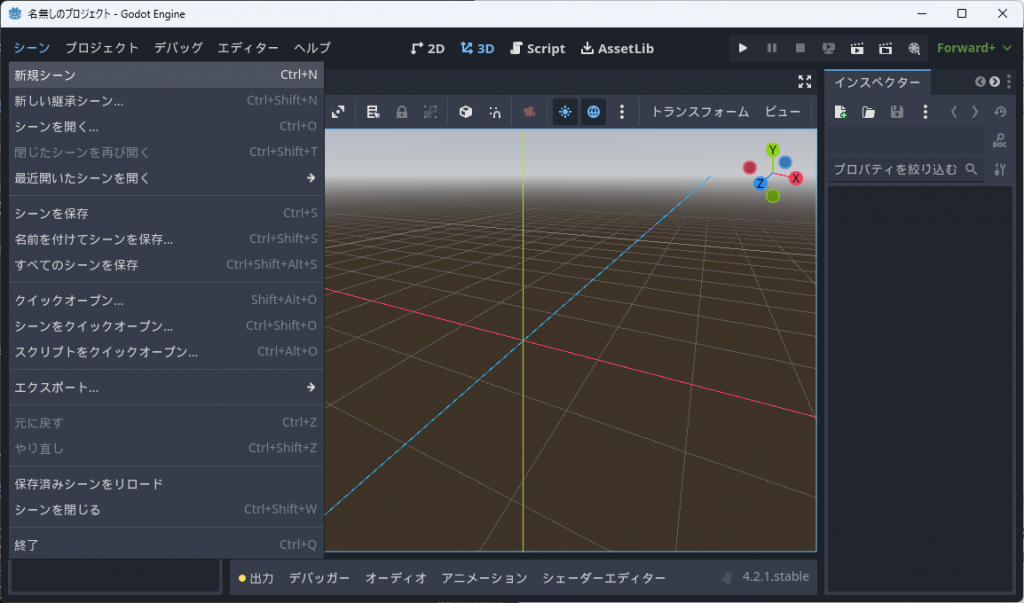
左ペインの「シーン」のタブに「ルートノードを生成:」という欄が現れるので、「その他のノード」を選ぶ。
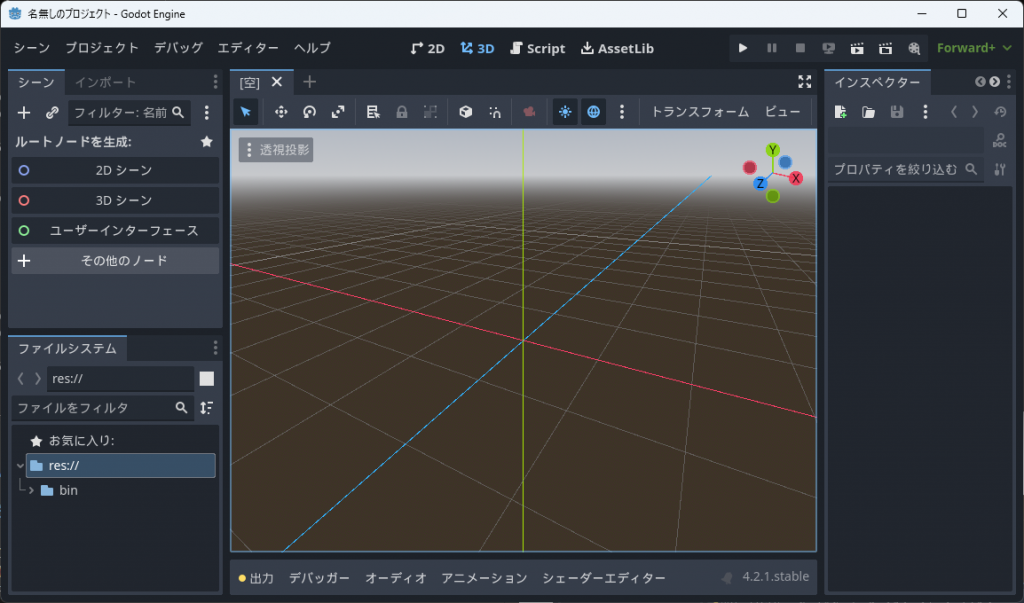
検索用のノードツリーが現れるので、「Node2D」を選択し、下にある「作成」を押下する。
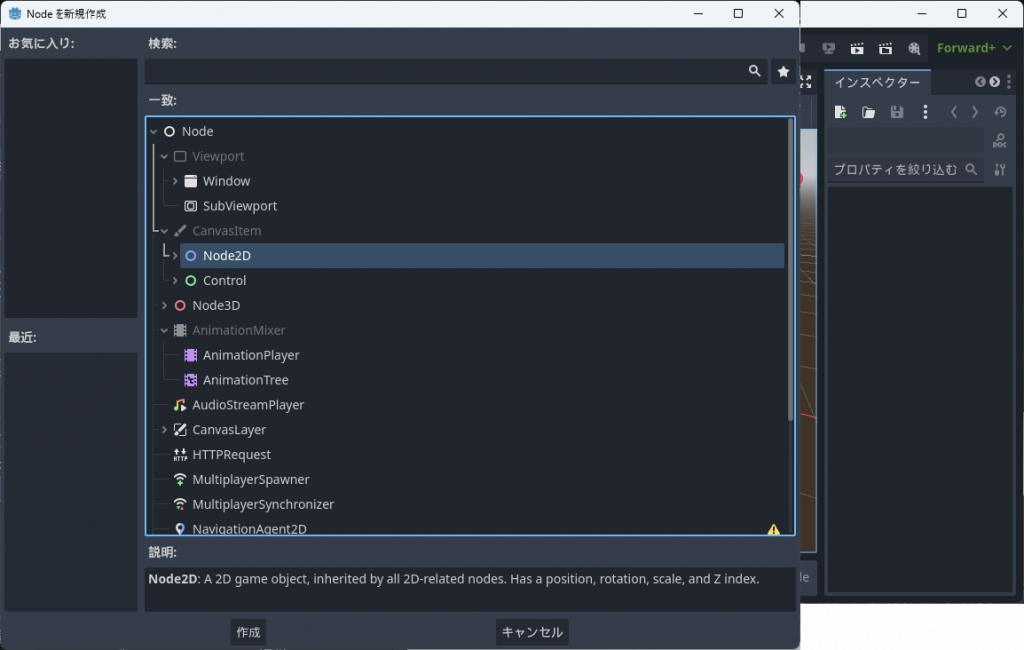
画面が 3D 表示から 2D 表示に切り替わる。
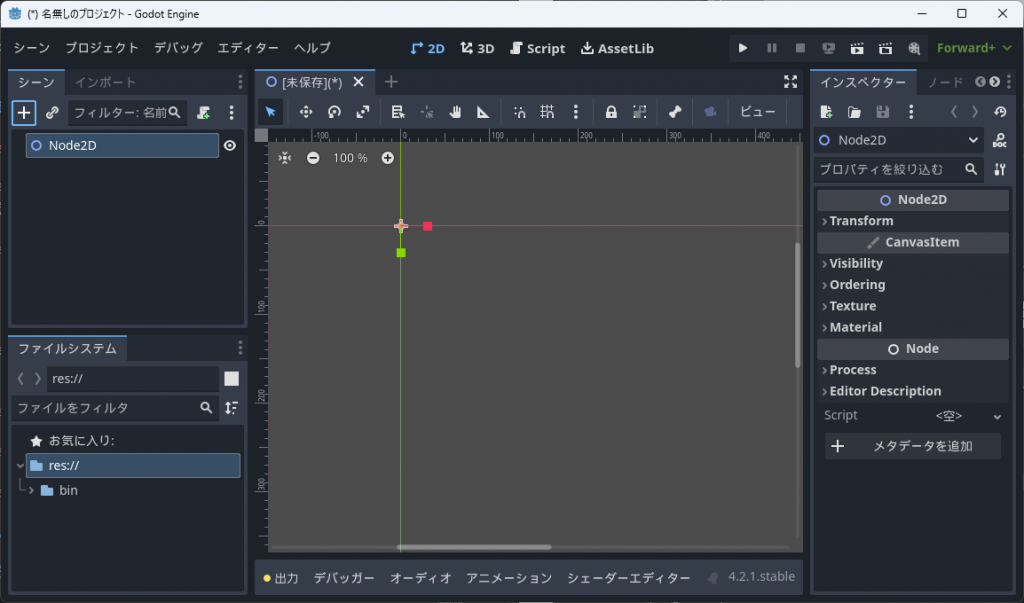
ここで左ペインの「シーン」のタブ直下にある「+」を選択するとメニューが現れるので、「子ノードを追加」を選択する。
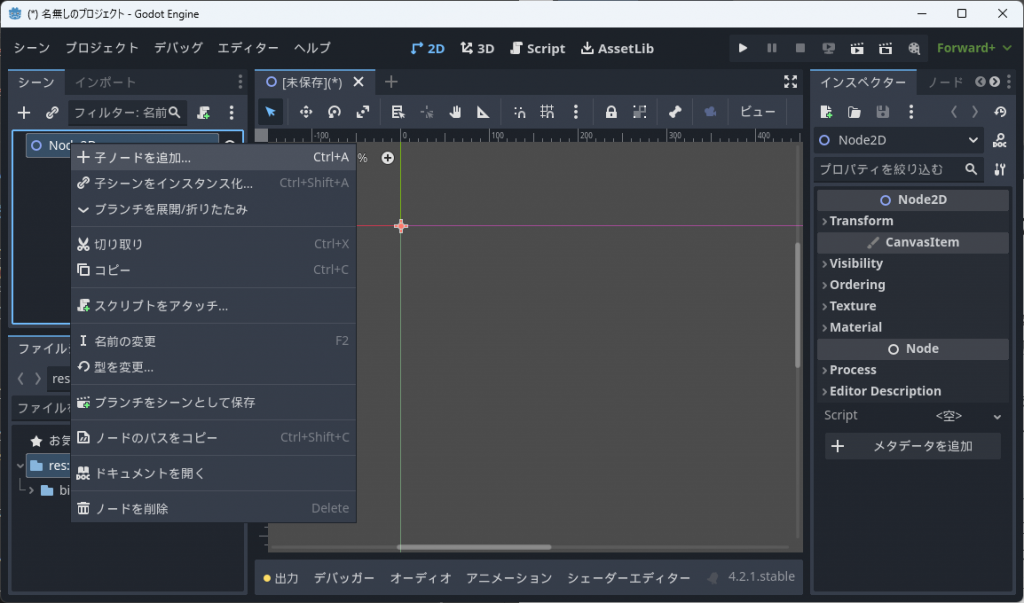
検索用のノードツリーが現れるので、検索欄に “gdexample” と入力すると、”GDExample” というノードが現れるのが期待値。
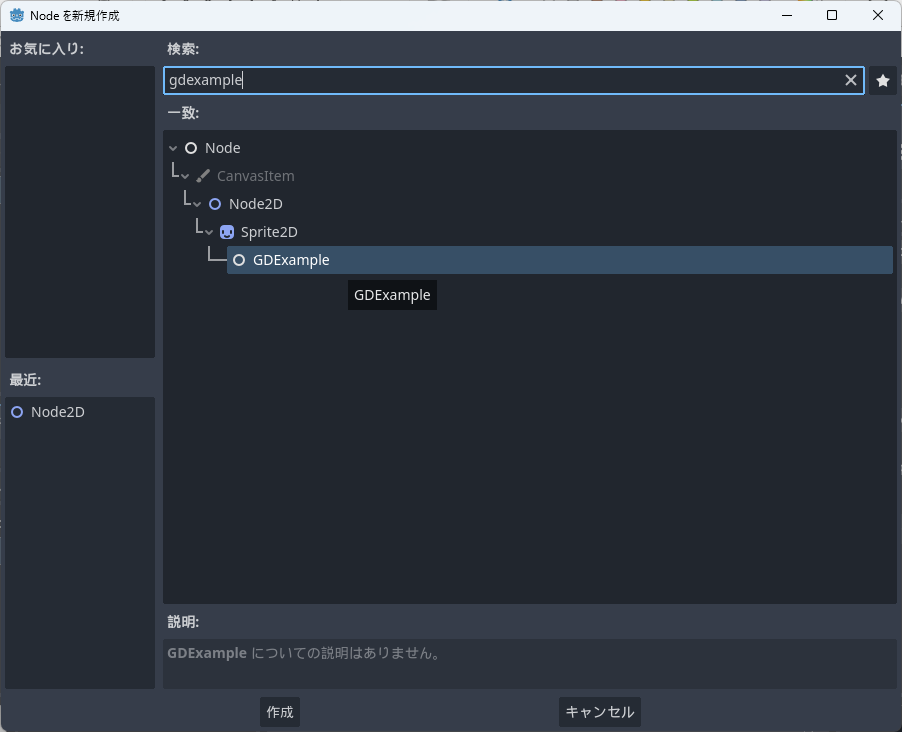
“GDExample”は Splite2D のプロパティを持っているので、右ペインのインスペクターでTexture を設定することができ、設定した画像が自動で動く様が確認できる。
ここでは icon.svg を選択する。
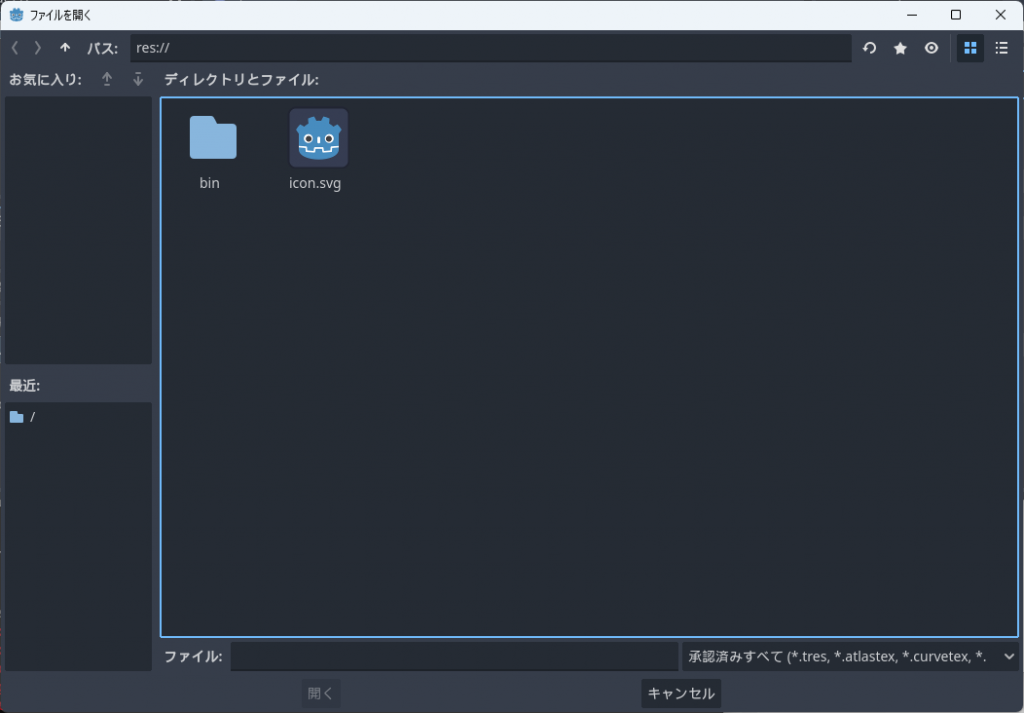
Godot のキャラクターアイコンが動けば Windows 用デバッグビルドの GDEXTENSION が正常に動作している。
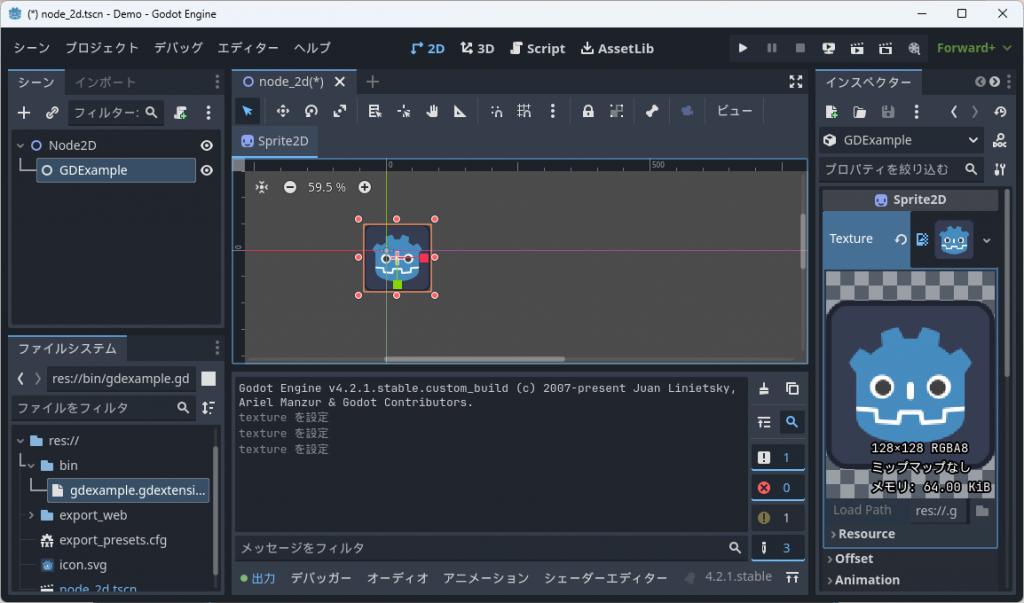
Ctrl-“S” とし、このシーンを ”node_2d.tscn” として保存する。
上部メニューの「プロジェクト」の「プロジェクトの設定」を選択すると「プロジェクトの設定」が開く。「一般」の「実行」でメインシーンを “res://node_2d.tscn” に設定する。
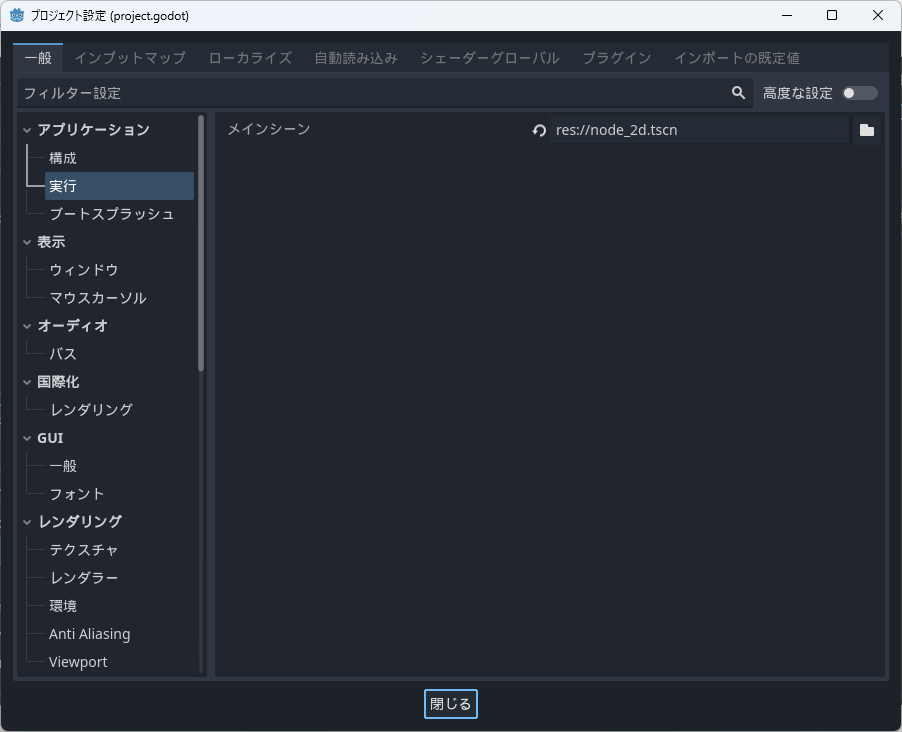
設定が終わった後 「F5」を押下すると、メインシーンが実行される。特に調整していないので、左上で奥ゆかしく動いている。
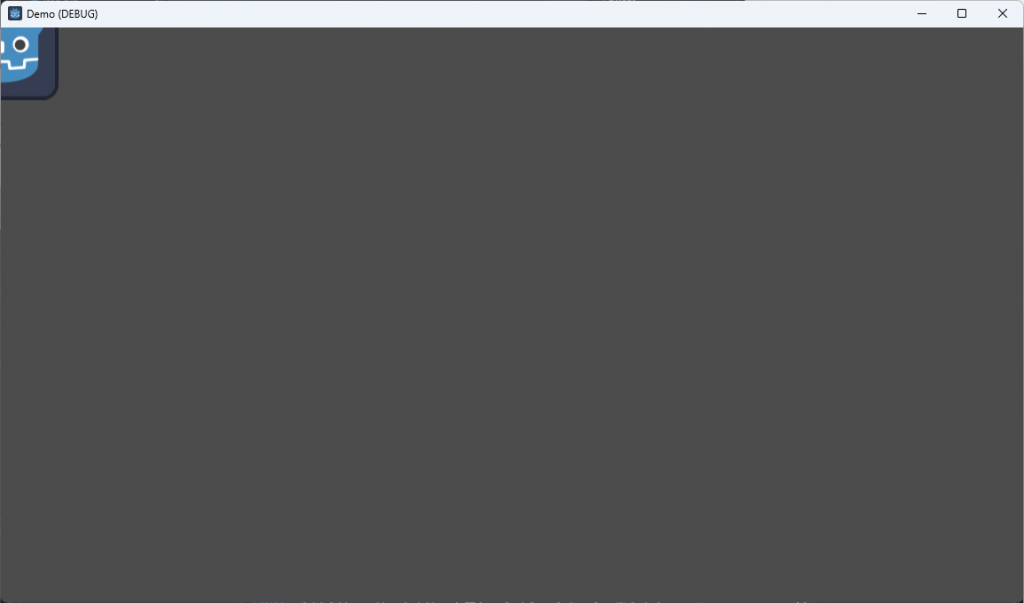
WEB エクスポート
上部メニューの「プロジェクト」の「エクスポート」を選択すると「エクスポート」のダイアログが開くので、「追加」を押下して「Web」を選択すると左ペインで「Web(実行可能)」が選択できるようになる。「Web」を選択すると右ペインに設定が表示される。
「エクスポート先のパス」を設定する。
ここでは、”c:/dev/demos/export/demo_05/web” というディレクトリを作成し、そこに “Sample.html” というファイルとして出力することにする。また、「バリアント」を “Extensions Support” のチェックボックスを ”オン” にする。
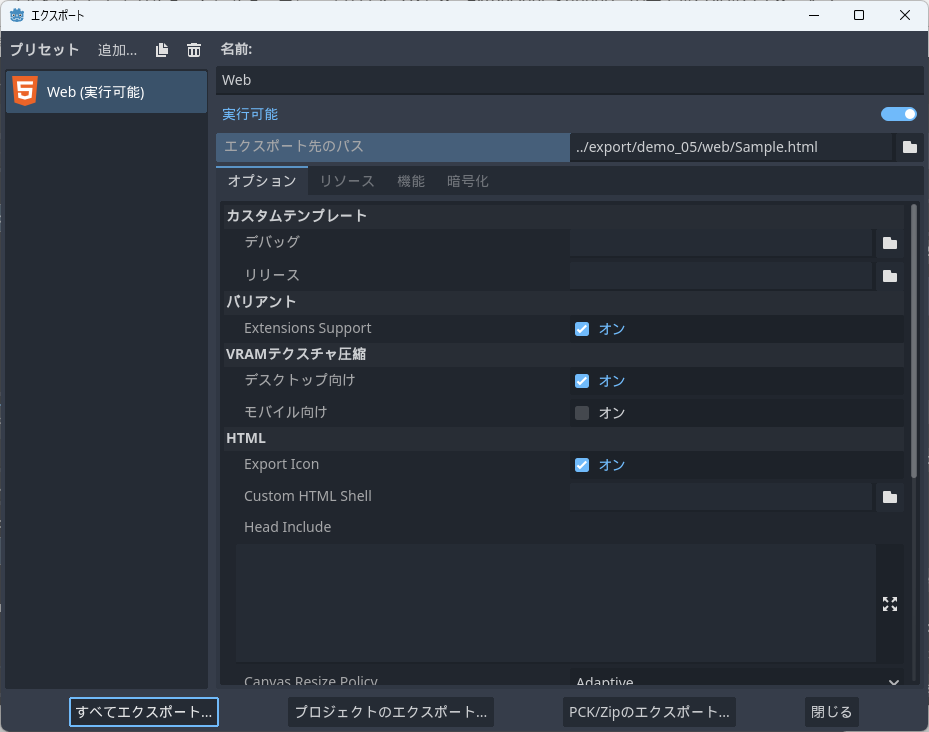
左下にある「全てをエクスポート」をマウス左クリックすると、「全てのエクスポート」ダイアログが開く。
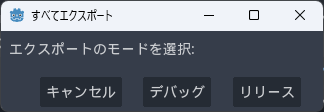
ここで、「デバッグ」を選べばデバッグ用にエクスポート、「リリース」を選べばリリース用にエクスポートされる。
c:\dev\demos\export\demo_05\web>dir ドライブ C のボリューム ラベルは Windows です ボリューム シリアル番号は 7C92-75B3 です c:\dev\demos\export\demo_05\web のディレクトリ 2024/02/06 12:23 <DIR> . 2024/02/06 12:21 <DIR> .. 2024/02/06 12:23 851,549 libgdexample.web.template_debug.wasm32.wasm 2024/02/06 12:23 12,190 Sample.apple-touch-icon.png 2024/02/06 12:23 7,199 Sample.audio.worklet.js 2024/02/06 12:23 6,583 Sample.html 2024/02/06 12:23 5,578 Sample.icon.png 2024/02/06 12:23 3,259,816 Sample.js 2024/02/06 12:23 5,824 Sample.pck 2024/02/06 12:23 21,443 Sample.png 2024/02/06 12:23 53,518,703 Sample.side.wasm 2024/02/06 12:23 2,866,393 Sample.wasm 2024/02/06 12:23 5,634 Sample.worker.js 11 個のファイル 60,560,912 バイト 2 個のディレクトリ 110,779,260,928 バイトの空き領域 c:\dev\demos\export\demo_05\web>
「リリース」の場合は以下。
c:\dev\demos\export\demo_05\web>dir ドライブ C のボリューム ラベルは Windows です ボリューム シリアル番号は 7C92-75B3 です c:\dev\demos\export\demo_05\web のディレクトリ 2024/02/06 12:27 <DIR> . 2024/02/06 12:21 <DIR> .. 2024/02/06 12:23 851,549 libgdexample.web.template_debug.wasm32.wasm 2024/02/06 12:27 851,699 libgdexample.web.template_release.wasm32.wasm 2024/02/06 12:27 12,190 Sample.apple-touch-icon.png 2024/02/06 12:27 7,199 Sample.audio.worklet.js 2024/02/06 12:27 6,585 Sample.html 2024/02/06 12:27 12,190 Sample.icon.png 2024/02/06 12:27 3,259,820 Sample.js 2024/02/06 12:27 5,824 Sample.pck 2024/02/06 12:27 21,443 Sample.png 2024/02/06 12:27 38,990,401 Sample.side.wasm 2024/02/06 12:27 1,758,196 Sample.wasm 2024/02/06 12:27 5,634 Sample.worker.js 12 個のファイル 45,782,730 バイト 2 個のディレクトリ 111,337,783,296 バイトの空き領域 c:\dev\demos\export\demo_05\web>
一応設置してみた。ディスクトップの Windows PC で Chrome ブラウザを用いているのなら、再生できると思う。
This sample is using godot engine and from GDExtension C++ example in GDExtension C++ example. License note is in https://godotengine.org/license/.
本稿の振り返り
最小構成であるが Windows ターゲットの GDEXTENSION サンプルが Web ブラウザでも簡単に動作した。全く同じ C/C++ のソースコードがクロスプラットフォームで動作することは非常に興味深い。
emscripten による WEB アプリケーション開発/デバッグは非常に面倒くさいものがある。なので有用性は認めるものの、あまり突き詰めて考えてこなかった。今回 Windows アプリケーションとしてのビルド環境と、WEB アプリケーションとしてのビルド環境を構築しできたので、Windows アプリとして動作確認したものを WEB 向けにビルドするといった使い方ができる可能性がでてきた。
次稿では、emscripten ターゲットの C++ ライブラリのビルドを試みる。
コメント